Tasty Data
I like to explore the free offerings of the airplane's WIFI when flying. It helps pass the time.
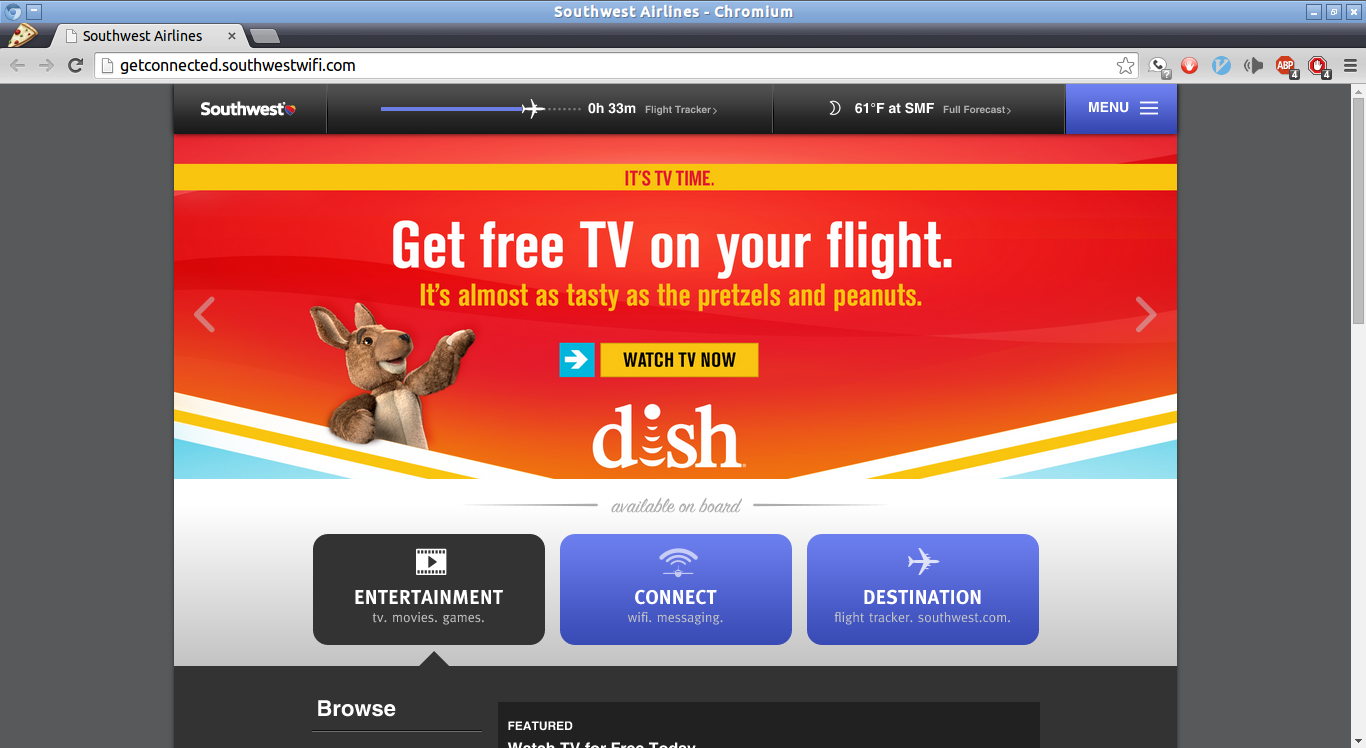
On a recent flight to San Diego, I noticed a banner that gives various information about the flight.
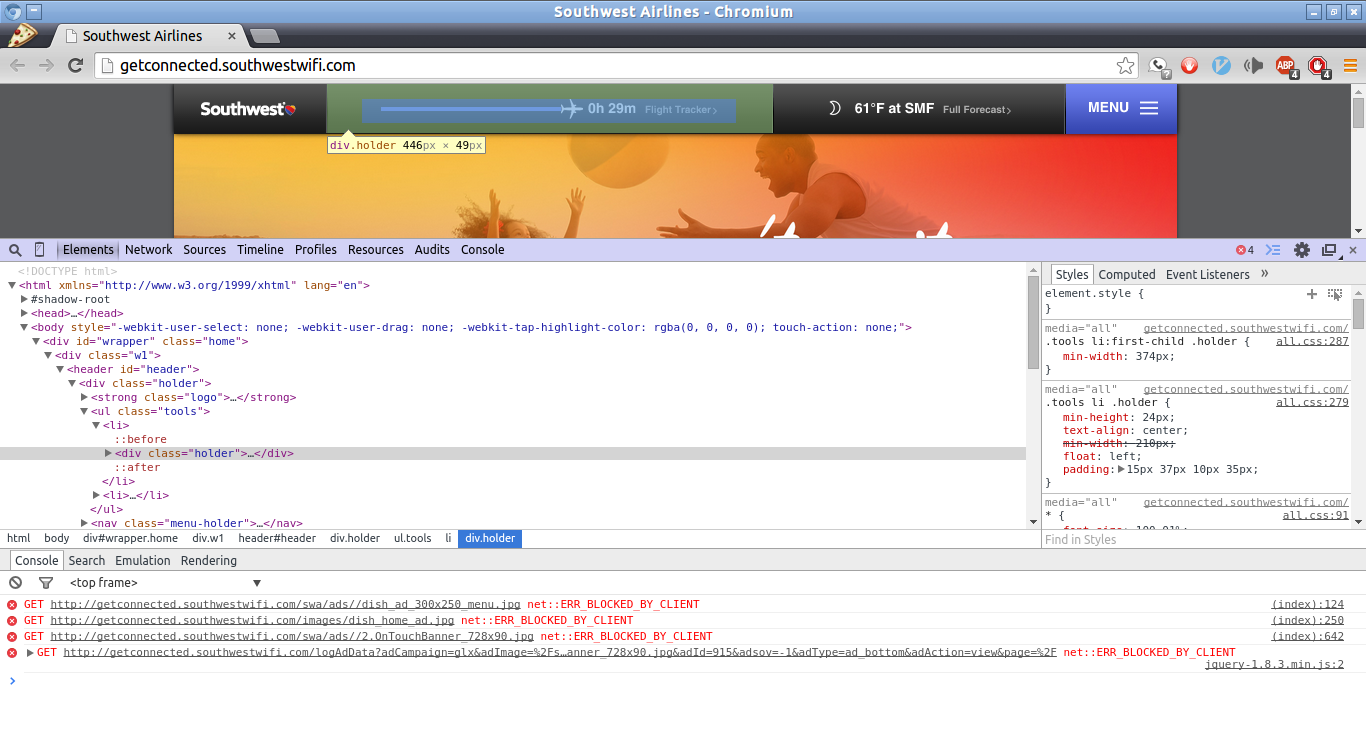
I knew the data was coming from some where but not exactly where. The Chromium inspector tool helped identify the source.
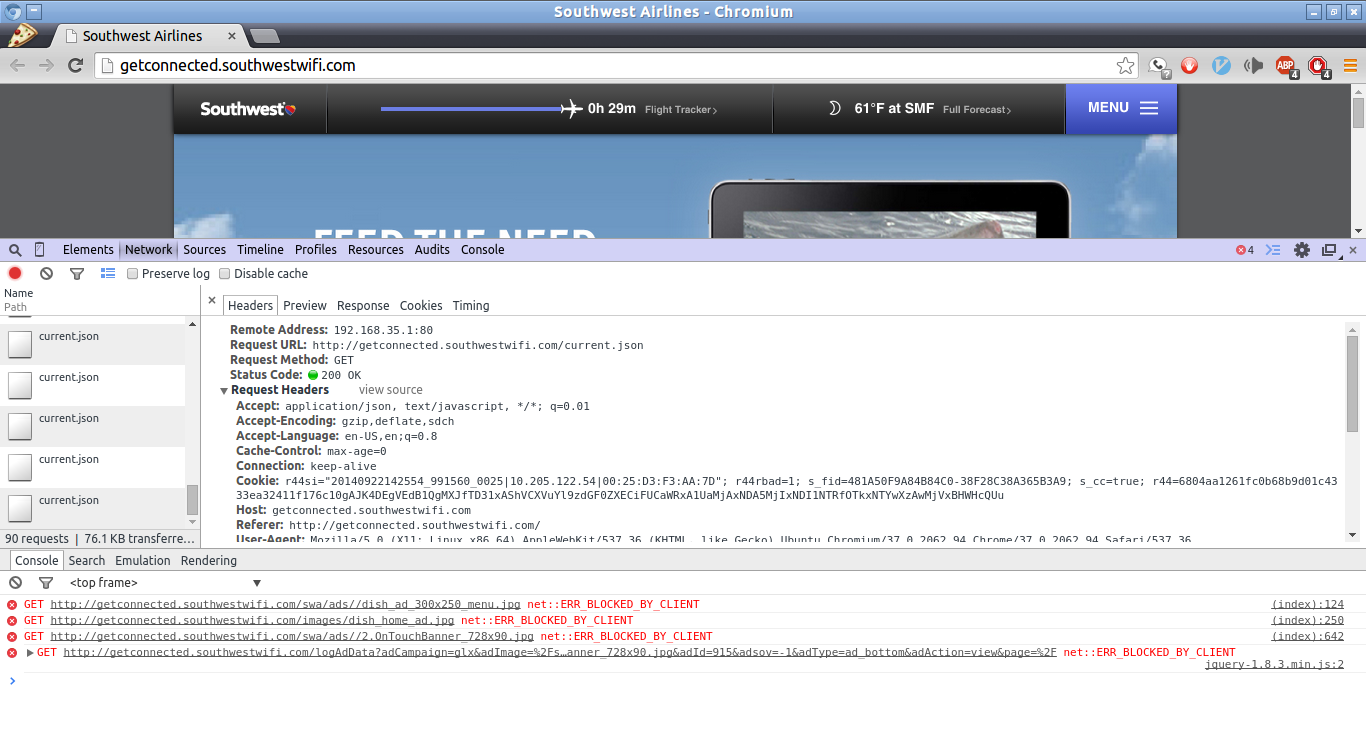
The flight data is formatted in JSON so it will be easy to parse.
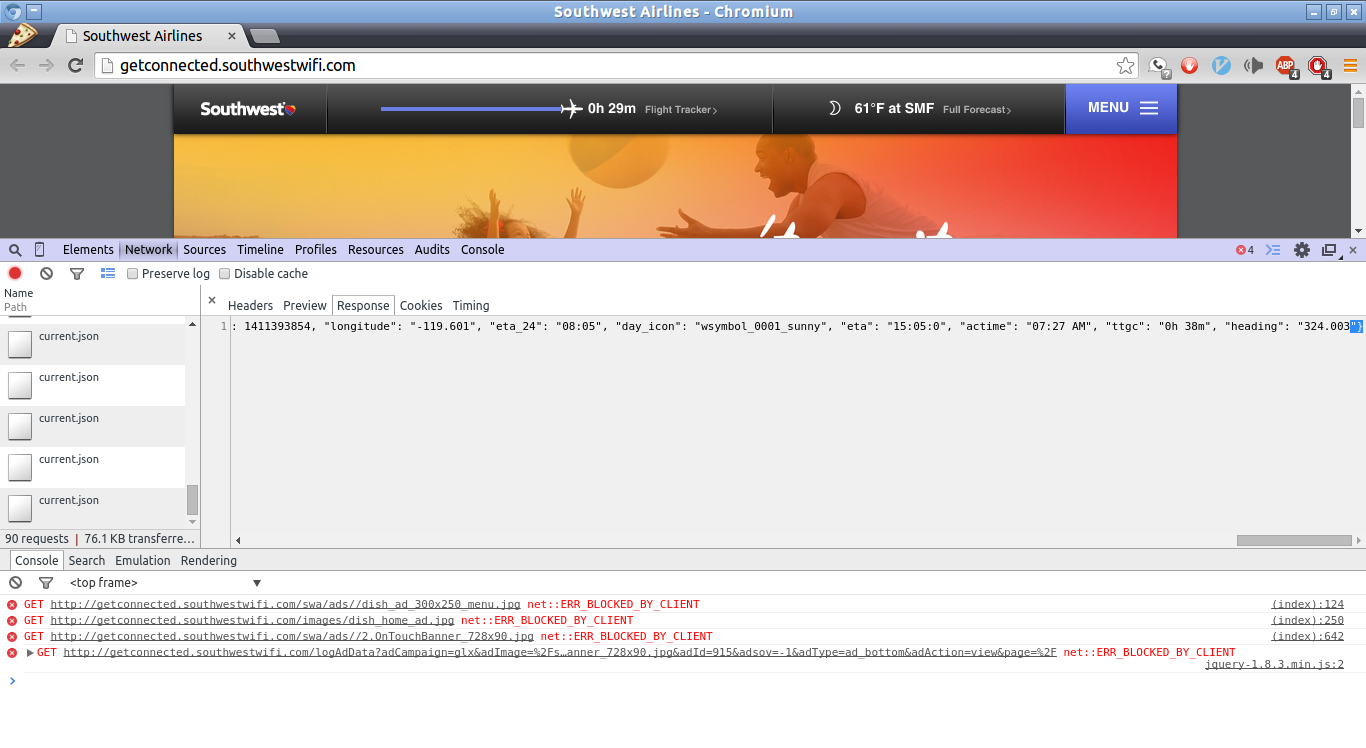
Data Acquisition
I put together a quick bash scrip to collect the flight data at a regular interval.
╰─➤ cat Sandbox/flight/flight.sh
#!/bin/bash
#script to fetch flight info JSON at a regular interval
while ((1 == 1));
do
wget http://getconnected.southwestwifi.com/current.json
sleep 10
done
I choose to run the script at a 10s interval to ensure that I was not being disruptive. Once the data was collected it was ready for processing.
╰─➤ ls Sandbox/flight/
current.json current.json.155 current.json.211 current.json.44
current.json.1 current.json.156 current.json.212 current.json.45
current.json.10 current.json.157 current.json.213 current.json.46
current.json.100 current.json.158 current.json.214 current.json.47
current.json.101 current.json.159 current.json.215 current.json.48
current.json.102 current.json.16 current.json.216 current.json.49
current.json.103 current.json.160 current.json.217 current.json.5
current.json.104 current.json.161 current.json.218 current.json.50
current.json.105 current.json.162 current.json.219 current.json.51
...
Next a VERY crude python script was written to parse the flight data. I am not proud of it but it worked surprisingly well and was developed at 40,000 ft with no internet.
╰─➤ ls Sandbox/flight/
#!/usr/bin/python2
#lazy script to parse out some information
import sys
json= ""
with open(sys.argv[1]) as fp:
for line in fp:
line = line.strip('\r\n')
json+= line + "\n"
print json[45:70]
╰─➤ for f in $(ls -C current*); do; ./flight.py $f; done > altitude.csv
I will only demonstrate altitude here but there is all sort of data in the JSON file including time, latitude, longitude, ground speed, heading etc.
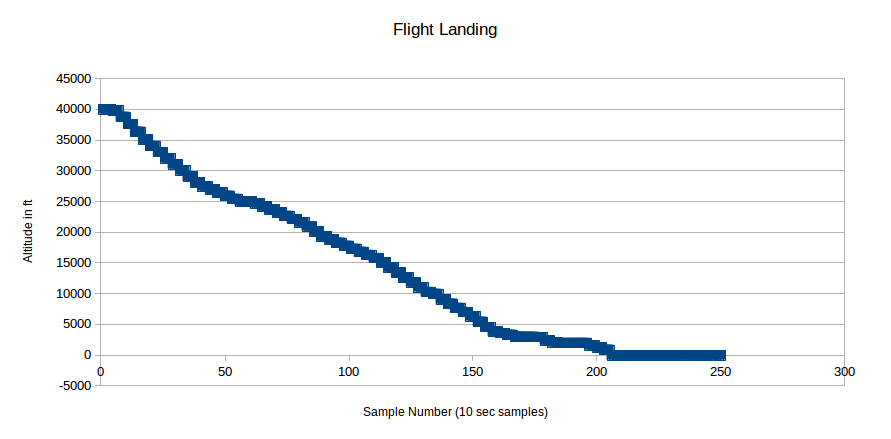
╰─➤ cat current.json
{"orig_code": "SAN", "headingAbbrStr": "NW", "altitude_f": "40 004 ft", "altitude_m": "12 193 m", "image_white": "night_white.png", "gspeed_m": "505 mph", "wth_tempf": "61", "ttg": "179", "lochist": [["32.733556", "-117.189667"], ["32.772", "-117.276"], ["33.026", "-117.598"], ["33.469", "-118.193"], ["33.629", "-118.320"], ["34.118", "-118.540"], ["34.602", "-118.755"], ["34.784", "-118.837"], ["35.025", "-118.946"], ["35.086", "-118.973"], ["35.146", "-119.000"], ["35.206", "-119.028"], ["35.265", "-119.055"], ["35.563", "-119.191"], ["35.681", "-119.245"], ["35.740", "-119.272"], ["35.974", "-119.389"], ["36.083", "-119.459"], ["36.193", "-119.530"], ["36.248", "-119.565"], ["36.357", "-119.637"], ["36.428", "-119.683"], ["36.482", "-119.718"], ["36.538", "-119.754"]], "gspeed_k": "813 km/h", "dest_code": "SMF", "gspeed": "505", "wth_tempc": "16", "altitude": "40004.625", "currentF": "61\u00b0F at SMF", "dtzone": "PDT", "currentC": "16\u00b0C at SMF", "current": 1411396199, "headingStr": "Northwest", "tail": "N7728D", "night_icon": "wsymbol_0008_clear_sky_night", "latitude": "36.538", "dist_remain": "179", "image_gray": "sunny_gray.png", "image_purple": "sunny_purple.png", "flight": "1008", "description": "Sunny", "pcent_flt_complete": 62, "wth_condition": "Clear", "dest_city": "Sacramento", "eta_utc": 1411398332, "wth_icon": "", "orig_city": "San Diego", "eta_ampm": "08:05 AM", "condition": "Clear/Sunny", "fltleg": "1", "success": true, "offgnd": 1411393854, "longitude": "-119.754", "eta_24": "08:05", "day_icon": "wsymbol_0001_sunny", "eta": "15:05:0", "actime": "07:29 AM", "ttgc": "0h 36m", "heading": "324.031"}%